While preparing for interviews for Amazon, Facebook and Google i came across a lot of resources. So here is a little collection that worked for me and some thoughts :)
First Steps
Do you know what you want/makes you happy on your next job?Draft a Wish list, this will help you position yourself. For me, for examples somethings always make the top: Work with Smart people, High availability and Big Scale, Being valued, TRAVELLING... and so on.
Do you know where you would like to be at within 2 years? .. and within 5 years?
This is important, you might not know or even care as all you can think of is: i just want to get a job. Why is it important? At a personal level goals change all the time and you might get it wrong but that is ok, use agile and re adapt. Makes it easy for you and for a company trying to hire you if you have some idea of where you want to be (.. even if it changes in a month). Another aspect of this is that this will help you structure your questions and understand if a particular company has the path you want. For me it's always important that the company has a easy way to swap teams or even job family because i know that after a while i will want it. I have been a Software Manager for the past 4 years (almost) and although i want to maintain myself technical I also want to learn how to manage managers. I have done it only once and for a brief time and i know i have made a lot of mistakes, will this new company help me grow on this area? Will i have the opportunity to work with peers that will mentor me and have a manager that will groom me towards it?
Also important for me is the opportunity to learn new skills, I want to learn more about Machine Learning, might not even be within the scope of my team but still.. Again i will ask if this company will give me this opportunity.
I don't know which position you are applying for or which company but whatever it is i am sure you can make this mental revision and if you can't you can always put down: Will this environment/company will help me define my path and understand better what i like? This can translate into a good career management and flexibility to try out new things so you can discover passions.
Structure your study
Well as in anything you need a bit of discipline or procrastination will creep in and you will tend to go and do exercises you feel comfortable with or re read things you already know.. and then you end up just watching another episode of Big Bang Theory :D
Here is a list of things you should master if this is a software engineer position:
Technical (this fits a generic Software engineer Role)
This is what is normally expected of a mid-senior engineer and covers coding and also Algorithms and Data Structures questions.
- Be familiar with at least one language. What does this mean? You should know most of the typical api's (e.g. in Java if I want to use Iterator i should know the interface)
- Object Orientated Design and Programming. Make it SOLID and know Design Patterns :). If you are not comfortable take a look into the Read section. A Really good book would be GangOfFour but might be easier to go through some of the videos on youtube (e.g. Code Walks).
- Testing. More than any fancy framework/library you should be well versed on what edge cases are necessary (e.g. test empy, max, min, duplicates, etc)
- Algorithms. Know how to approach the problem with bottom-up and the top-down algorithms. You should know the complexity of an algorithm and how you can improve/change it. tipycal algorithms that are used to solve problems include: sorting (plus searching and binary search), divide-and-conquer, dynamic programming/memoization, greediness, recursion or algorithms linked to a specific data structure.
- Know Big-O notations (e.g. run time, space) and be ready to discuss standard, well established algorithms. You may wish to discuss or use bullets to outline the algorithm you have in mind before writing code. Love this Big-O Cheat Sheet, memorising is not the answer but this helps pin point when you look into an algorithm.
- Sorting: Be familiar with common sorting functions and on what kind of input data they’re efficient on or not. Think about efficiency means in terms of runtime and space used. For example, in exceptional cases insertion-sort or radix-sort are much better than the generic QuickSort/MergeSort/HeapSort answers.
- Data structures: Study up on as many other structures and algorithms as possible. Read about the most famous classes of NP-complete problems. You will also need to know about trees, basic tree construction, traversal and manipulation algorithms, hash tables, stacks, arrays, linked lists, priority queues, and when they are appropriate.
- Recursion: Many coding problems involve thinking recursively and potentially coding a recursive solution. Prepare for recursion—which can sometimes be tricky if not approached properly. Use recursion to find more elegant solutions to problems that can be solved iteratively.
- Mathematics: Some interviewers ask basic discrete math questions. Spend some time before the interview refreshing your memory on (or teaching yourself) the essentials of elementary probability theory and combinatorics. You should be familiar with n-choose-k problems and their ilk.
- Scale & Design
- On Coding - does your algorithm/DS scale if it's used by 1 billion users? You should be able to adapt and flush stored memory according to the given scale or at least walk the interviewer through the thought process of it
- On Systems - At least for me this one comes with experience and as a mash up of a lot of things and becomes intuitive. But good designs follow some principles and you should know them. Here is a good lecture :)
Management/Leadership
Different companies have different management styles and expectations but all off them will cover Performance Management, Work frameworks(Scrum, Kaban, etc) and Career Development.
Google, Facebook and Amazon will focus on team delivery from different perspectives. Google will focus on Impact either internally or externally. Facebook approaches things on building fast and failing fast again the Delivery is important. Amazon likes to Deliver Results and focus on customer impact, this is very important if you want a job at Amazon, make sure you know all Leadership Principles.
http://steve-yegge.blogspot.co.uk/2008/03/get-that-job-at-google.html
Read

Job Descriptions
- Read your Job description :) Identify what you feel and don't feel comfortable with, map it and try to mitigate it.. or just be honest about it with the recruiter to understand if it's ok to have those gaps. I applied to SRM and i was very honest that my strength is in Software Engineering and not so much on infrastructure, it would be impossible for me to learn it in a couple of weeks and that was fine.
- SRE book link (part 4 is around management)
- Cracking the Coding Interview
- GangOfFour - For Object Oriented Programming
- Introduction to Algorithms
- If you are Java geek :D Effective Java
Online Resources and Examples
- "Solving the most interesting problems"
- Problem Solving with Algorithms and Data Structures using Python
- High Scalability (great to practice for design interviews)
- Software Engineering Advice from building Large Scale Systems, Jeff Dean Video: Building Software Systems At Google and Lessons Learned (Most Important)
Practice!
Coding
- LeetCode: https://leetcode.com/
- HackerRank: https://www.hackerrank.com
- TopCoder www.topcoder.com
- Project Euler http://projecteuler.net/
- Why not code most of the commonly known datastructures (Binary Tree, Tries, etc) and algorithms(MergeSort, QuickSort, etc) yourself. Create your library. This will help you remember a lot of implementation patterns and algorithms.
- If you don't use TDD, try it. Or at least use some unit testing before submitting your exercises. This will keep you on top of how to get Edge cases. I like LeetCode as they normally have a great suite of tests (Yeah i do miss some edge cases.)
- For code style: Google Style Guide
- Do little breaks to listen to youtube and understand more deeply concepts or just to review i had forgotten that Tries existed.. bad me). It will program your brain. Here are some resources:
- GeeksforGeeks channel
- HackerRank channel ( from Gayle Laakmann McDowell author of Cracking the code Interview, it's a really neat book)
- INFRASTRUCTURE & OPERATIONS - How Google Does Planet-Scale Engineering for Planet-Scale Infra - Talk by Melissa Binde - Site Reliability Engineering
- My Code School
- There is also Treehouse and Plurasight (pluralsight is a bit more towards .net but nevertheless it has great foundations)
- Design Patterns
- Be prepared for technical questions involving coding algorithms and data structures. Normally you can code in any language you wish.
- Consider things like memory constraints, the complexity of the algorithm you are writing (and its running time O(N^2) to O(N) etc.) and how your solution can be improved and optimised.
- Produce clean, efficient code in a reasonable amount of time and be able to optimise your solution. You should also check for edge cases and test your code to ensure it is as bug free as possible.
- It may help to review core CS concepts (data structures, binary trees, linked lists, object oriented analysis/design, design patterns etc.) as well as subjects pertaining to the scale of our environment.
- Always make sure you understand your input/output as it might be key to your problem solving: if it's a string what charset is it, what encoding,; if it's a number is it an integer, can it go bellow zero and so on; is it a set?
- It is recommended to practice solving problems on a whiteboard or a piece of paper in front of a friend who can help you spot bugs and so you're comfortable problem solving out loud - Always start with the top left corner and test your pens hehehehe.
Design
Designing solutions for very large scale distributed system/sub-system (think of scalability, data centers, network latency, redundancy, concurrent users, etc.) With this interview, thinking of scale is KEY. also try and think of the most optimal design and trade-off. Be sure to ask clarifying questions, suggest alternatives, and propose better solution. It may be helpful to think of it as a technical discussion with a colleague where the objective is to come up with a design document for a new system. Refresh your knowledge or learn about *numbers and orders of magnitude of Internet, computers and networks*. That means for instance to have some basic ideas about number of internet users, the time they spent on internet, size of network packets. RTT on Internet... and also about time taken for various basic computer operations (cpu cache access, memory access, disk access, network data transfer etc. Knowing how things work from the outside is not enough, you have to understand how they have been implemented (and better why it is this way!). Review your existing knowledge from that perspective. For example, don't think you know map/reduce if you don't know what in-memory and on-disk data structures are used, how data are transferred and what are the tradeoffs made in some map/reduce implementation Be ready to quickly do back-of-the-envelope estimates. You may want to play some estimate games (search fermi problems) to be at ease with that.Some example questions are: Design a key-value store Design Google search
Architect a world-wide video distribution system Build Facebook chat
Build Instagram
They may focus on some machine learning, networking system, iOS or JavaScript if you have that domain expertise on your resume. They don't expect you to know crazy algorithms that you likely wouldn’t know off the top of your head (like quadtrees or Paxos). The emphasis is on how you perceive the system and problem space.
Make sure you understand concepts like sharding, fan-out, partitioning, batching, queuing, etc
Some tips on how to tackle it:
- Write down all use cases, make sure you understand what is expected of this system you are designing
- Write down the dimension of the problem, if there are inputs what are the size, how many per second, what is the format.
- Is it global? Ask if you need data consistency or if it can be eventually consistent. Ask if you need to have security considerations?
- What are you optimising for on each use case?
- Remember single responsibility? Still applies so if you have the use cases start expanding from those. Example: I need something that parses a log so you have a component Parser :)
- Get a simplistic design make sure you understand the problem. Do not get into rabbit holes and get stuck in a specific component before having solved a working solution.
- Once you have a working solution.. Scale it to the size of the problem, be concrete. At Amazon they won't give you what kind of machine you have available but you can still check the dimension of the problem and go through it. At Google they give you specific numbers. This is almost like TDD, while sizing you will re-adapt your solution. Might not be feasible to have 6 TB of Data in Memory.
- Pay attention on single points of failure and how to cope with it. Your system needs to be reliable.
- Trade Offs: creating duplicate data might be worthwhile depending on what are you optimising for. I always remember the twitter architecture example.
- Create redundancy.
- Some interviews expect you to calculate how many machines will you need according to the specification as the ultimate result of the interview but i only got that at Google.
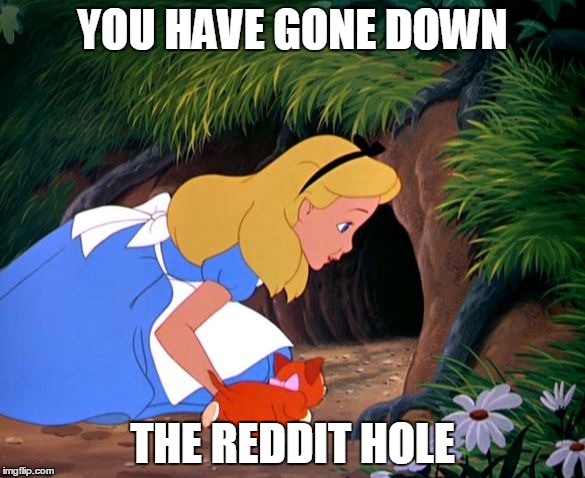
Management and Leadership
Slightly more complicated to practice but a good idea would be to go through examples that you went through and if you haven't try to role play scenarios.
You should have examples of: Bad Performance Management, Developing your Engineers, Conflict Management inside and outside the team, Change Management, Strategy Thinking: how to build a road map and challenges, Influence: How to influence Peers and Upper Leadership. How do you balance and negotiate priorities with stakeholders.
You will be given scenario like questions and can range on anything from performance management (example: you have a strong engineer underperforming, how do you get them back on track) or conflict resolution (example: designing a fair on-call rotation or you have two engineers who are conflicting with each other, how do you resolve it). You should always be thinking of retention for the staff and motivating them back to full effectiveness.
The questions will feel short but feel free to go in-depth for your answers while giving examples however it's important to stay on point as well.
At Amazon the questions will be less scenario driven and more example driven: Tell me about a time when you had someone on your team performing bad? These are still the same topics just approached on a slightly different manner. Facebook also goes more into actual examples then scenarios.
A Manager will also need to know about project management, normally this goes a lot into Agile methodologies but be expected to know how to react to waterfall and how do you plan accordingly. What metrics do you use on your team? Velocity? How do you use velocity? How do you estimate with the team? Tell me about a time when upper management gave you a date and a project to deliver and how did you went about it given that the time constraint was critical?
Some things you have learned intuitively so try to list down all the steps taken and read best practices on each topic.
Day 2 Day knowledge
Regardless of the interviews there are some day to day things you should know... if you don't.. pick it up pick, it up!
- Back of the Envelope Calculations
- Latency Values
- Data Measurements
A bit Before and On the day of the interview:
-- Before -- (day - 1)
Learn about the Company
Learn about the Company
Create a list on why would you want to work for this company.
Learn about the company and be a stalker, go the the linkedIn profiles of the interviewers, it will give you some insight on what
Overcome Impostor Syndrome
Listen/Read to what impostor syndrome is, how normal it is and just dismiss it:
https://blog.valbonne-consulting.com/2014/08/16/the-imposter-syndrome-in-software-development/
https://www.laserfiche.com/simplicity/shut-up-imposter-syndrome-i-can-too-program/
Sleep well!
Learn about the company and be a stalker, go the the linkedIn profiles of the interviewers, it will give you some insight on what
Overcome Impostor Syndrome
Listen/Read to what impostor syndrome is, how normal it is and just dismiss it:
https://blog.valbonne-consulting.com/2014/08/16/the-imposter-syndrome-in-software-development/
https://www.laserfiche.com/simplicity/shut-up-imposter-syndrome-i-can-too-program/
Sleep well!
Wake up early, do your morning routine without thinking too much about the interview.. just relax, put on some music, whatever makes you happy. (for me always includes expresso :D).
-- On the Day --
Start your engine! Before I go, I usually start the engine.. what do I mean by this.. your brain needs a kick to get it running. If i have interviews that require coding, typically, i get one of the easy coding exercises from leetcode, for example: https://leetcode.com/problems/valid-anagram/#/description and just smash it!
Start your engine! Before I go, I usually start the engine.. what do I mean by this.. your brain needs a kick to get it running. If i have interviews that require coding, typically, i get one of the easy coding exercises from leetcode, for example: https://leetcode.com/problems/valid-anagram/#/description and just smash it!
There is always commute time and you can make the best out of this time. Calm yourself and be confident of your skills, you know you know it. Review some things mentally and most and foremost Have Fun on your interview.
During the interviews. Take your time to understand the exercise, do as many questions as you want, whatever pops into your head just ask. There are no silly questions, don't be afraid of failure, not asking the question can be what trips you over and then you will regret not having done the question.
WRITE everything down. While you write your brain assimilates on a different level, even the smallest things. I create a little corner on the write board where i write all assumptions, my own doubts and answers. I also create a little corner for test cases.
Instead of speaking to yourself, speak out your thoughts, the interviewer won't interrupt you and it will be easier for him to follow where you are going. The interviewer was in your position once and he is now in the other side, this will make him nervous as for him it's a huge responsibility to evaluate you, empathise with him/her, he is on your side.
A good thing to keep in mind is: FAILURE == NOT TRYING. Most people don't even try to change jobs and are too afraid of being rejected. You got yourself this far, that is already success, if you are not accepted remember you tried, you are a step closer and you can always try again. The amount of false positives is really high and all companies know that. I know a lot of people that made it to Amazon after 2 attempts the same with Google and Facebook.
I have interviewed many people on my career and saw this a recurring thing. Being rejected does not imply that you are not good it implies that there wasn't sufficient data on the interview for the interviewers to feel confident on hiring, it is a risk for who is hiring. You can not expect human beings to get it right with 4 or 6 hours of interviewing. Also.. interviewers might be having a bad day :). So. TRY .. again and again and again until you Get what you Want.
Acknowledgements:
I have used resources that i got from Google, Facebook and Amazon when interviewing :) I should say i did work for Amazon Video and went through all 3 processes. [TBD if I have actually passed Google]
Sem comentários:
Enviar um comentário